|
Each entity of the main US map on click drills down to Detailed.asp page passing its Internal id and map swf file name to it. In this section we will see the code in Detailed.asp and how the page handle these requests and renders map. |
|
Before moving further with the process of plotting data from database, we recommend to go through the dataXML and dataURL method to know the basics about FusionMap generation procedure. |
|
The code discussed here is present in Download Package > Code > ASP > DB_Drill folder. |
The code of Detailed.asp file is as follows: |
|
<%@ LANGUAGE="VBScript" %>
<!-- #include file="../Includes/DBConn.asp" -->
<!-- #include file="../Includes/FusionMaps.asp" -->
<%
%>
<HTML>
<HEAD>
<TITLE>FusionMaps v3 - Database Example</TITLE>
<%
%>
<SCRIPT LANGUAGE="Javascript" SRC="../JSClass/FusionMaps.js"></SCRIPT>
</HEAD>
<BODY>
...
<%
dim strXML
strXML = strXML & "<map showCanvasBorder='0' borderColor='FFFFFF' connectorColor='000000' fillAlpha='80' hoverColor='FFFFFF' showBevel='0' legendBorderColor='F1f1f1' hoverColor='FFFFFF' legendPosition='bottom'>"
strXML = strXML & "<colorRange>"
strXML = strXML & "<color minValue='0' maxValue='93' displayValue='0%25 to 93%25' color='D64646' />"
strXML = strXML & "<color minValue='93' maxValue='94' displayValue='93%25 to 94%25' color='F6BD0F' />"
strXML = strXML & "<color minValue='94' maxValue='95' displayValue='94%25 to 95%25' color='8BBA00' />"
strXML = strXML & "<color minValue='95' maxValue='100' displayValue='95%25 or above' color='AFD8F8' />"
strXML = strXML & "</colorRange>"
strXML = strXML & "<data>"
strQuery ="select a.Internal_Id,a.entity_id,sum(data) as datap from fcmap_distribution a group by a.Internal_Id,a.entity_id having a.Internal_Id='" & request("Internal_Id") & "'"
set rs = Server.CreateObject("ADODB.Recordset")
rs.open strQuery,oConn
if rs.bof=false then
do while not rs.eof
strQuery ="select a.Internal_Id,a.entity_id,b.group_name,sum(data) as datap from fcmap_distribution a, fcmap_group_master b where b.group_id=a.group_id group by a.Internal_Id ,a.entity_id, b.group_name having a.Internal_Id='" & rs("Internal_Id") & "' and entity_id='" & rs("entity_id") & "'"
set rs2 = Server.CreateObject("ADODB.Recordset")
rs2.open strQuery,oConn
tooltext=""
totEmp=0
dim StateValue,TotalStateValue,StatePer
StateValue=0: TotalStateValue=0: StatePer=0
do while not rs2.eof
StateValue=cdbl(rs2("datap"))
TotalStateValue=cdbl(rs("datap"))
StatePer=round((StateValue / TotalStateValue) * 100,2)
tooltext = tooltext & rs2("group_name") & ":" & StatePer & "%25 \n"
if rs2("group_name") <> "Unemployed" then
totEmp = totEmp + StatePer
end if
rs2.movenext
loop
strXML = strXML & "<entity id='" & rs("entity_id") & "' value='" & totEmp & "' link='Charts.asp?" & server.URLEncode(request.ServerVariables("QUERY_STRING")) & "%26entity_id=" & rs("entity_id") & "' tooltext='" & tooltext & "' />"
rs.movenext
loop
end if
strXML = strXML & "</data>"
strXML = strXML & "<styles><definition><style type='animation' name='animX' param='_xscale' start='0' duration='1' /><style type='animation' name='animY' param='_yscale' start='0' duration='1' /><style type='animation' name='animAlpha' param='_alpha' start='0' duration='1' /><style type='shadow' name='myShadow' color='FFFFFF' distance='1' />"
strXML = strXML & "</definition><application><apply toObject='PLOT' styles='animX,animY' /><apply toObject='LABELS' styles='myShadow,animAlpha' /></application></styles>"
strXML = strXML & "</map>"
Call renderMap("../../Maps/" & Request("map"), "", strXML, "Map1Id", "750", "460", false, false)
%>
</BODY>
</HTML>
<%
set rs=nothing
set rs1=nothing
set rs2=nothing
oConn.close
set oConn=nothing
%> |
|
Let's discuss the process flow of this page in details: |
|
- Like the previous page we include FusionMaps.asp, DBConn.asp and FusionMaps.js.
- We also create a variable - strXML to store map XML.
- Next we initialize root map element and add color range settings in XML.
|
- Then using SQL we sum up the total population of each entity or county (represented by entity_id) of the selected state (represented by its Internal_Id).
|
"select a.Internal_Id,a.entity_id,sum(data) as datap from fcmap_distribution a group by a.Internal_Id,a.entity_id having a.Internal_Id='" & request("Internal_Id") & "'" |
|
- Now we iterate through each entity/county of the map/state and get details like percentage of employment in Business, in Service and percentage Unemployed population.
- To achieve this, we create another recordset rs2 using the following SQL:
|
select a.Internal_Id,a.entity_id,b.group_name,sum(data) as datap from fcmap_distribution a, fcmap_group_master b where b.group_id=a.group_id group by a.Internal_Id ,a.entity_id, b.group_name having a.Internal_Id='" & rs("Internal_Id") & "' and entity_id='" & rs("entity_id") & "'" |
- Here, rs2 stores records each having total of each group - Business, Service and Unemployed - in each record. So iterating through each, we calculate percentage of employment of each group and total employed population. This we did using the following calculation:
|
do while not rs2.eof
StateValue=cdbl(rs2("datap"))
TotalStateValue=cdbl(rs("datap"))
StatePer=round((StateValue / TotalStateValue) * 100,2)
tooltext = tooltext & rs2("group_name") & ":" & StatePer & "%25 \n"
if rs2("group_name") <> "Unemployed" then
totEmp = totEmp + StatePer
end if
rs2.movenext
loop |
- The above code also shows that we also generate custom tool-text for each entity to show Percentage of population in Business, Service and Percentage of Unemployed population for each district/entity of that state's map. We create a variable - tooltext and accumulate group name and corresponding percentage of each group in it.
- Moreover we also keep a total of employment percentage in totEmp variable. This we did by skipping "Unemployed" group.
|
|
Providing map data |
Let's now discuss how to provide data to each enity of the map. In the above outer iteration through each entity we also add our code to add <entity> element. |
|
strXML = strXML & "<data>"...strXML = strXML & "<entity id='" & rs("entity_id") & "' value='" & totEmp & "' link='Charts.asp?" & server.URLEncode(request.ServerVariables("QUERY_STRING")) & "%26entity_id=" & rs("entity_id") & "' tooltext='" & tooltext & "' />"
...strXML = strXML & "</data>" |
- As entity id we use the "entity_id" field from the first recordset - rs.
- We give total employed percent stored in totEmp as value attribute of each entity.
- We set tooltext attribute from tooltext variable.
- We also create links that drill-down to another page showing details of the clicked district/entity in charts.
|
Thus, we create entity data. |
|
- Finally we apply style like XScale, YScale, alpha & shadow styles to the map using <styles> element and
- Render the map using renderMap() function from FusionMas.asp.
- Request("map") gives the map swf name.
|
Call renderMap("../../Maps/" & Request("map"), "", strXML, "Map1Id", "750", "460", false, false) |
|
Here is the snapshot of the detailed county map: |
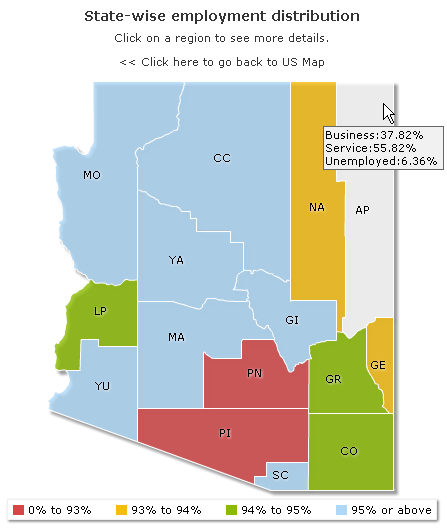 |