|
In this section we will see how to render a map using dataURL method - where the XML data is created in a file other than what we use to generate the map. The page that contains the code to render the map is referred to as Map Container Page and the other one which creates the XML data is called Data Provider Page.
Let's look at the codes used in Map Container Page and Data Provider Page one by one. |
|
Before proceeding further, we recommend to go through the documentation "How to use FusionMaps" for a better insight. |
|
All code discussed here is present in Download Package > Code > VB_NET > BasicExample folder. |
|
Map Container Page contains the following code. You can view this code in BasicMapsArrayURL.aspx file. |
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="BasicArrayURL.aspx.vb" Inherits="BasicArrayExample_dataURL" %>
<html>
<head>
<title>FusionMaps Sample - Basic Example from array - Using dataURL</title>
<script language="Javascript" src="../JSClass/FusionMaps.js"></script>
</head>
<body>
<form id='form1' name='form1' method='post' runat="server">
<% %>
<asp:Literal ID="WorldPopulationMap" runat="server" />
</form>
</body>
</html> |
|
In the above code, we first include FusionMaps.js file to enable us embed the map using JavaScript. The code behind script generates the map in the litreral control WorldPopulationMap. |
Let us see how code behind script in BasicMapsArrayURL.aspx.vb builds the map XML and renders the map: |
Imports InfoSoftGlobal
Partial Class BasicArrayExample_dataURL
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal ob As Object, ByVal e As EventArgs) Handles Me.Load
Dim dataURL As String
dataURL = "WorldPopulationData.aspx"
Dim mapHTML As String = FusionMaps.RenderMap("../Maps/FCMap_World8.swf", dataURL, "", "mapid", "600", "400", False, False)
WorldPopulationMap.Text = mapHTML
End Sub
End Class
|
|
- We include InfosoftGlobal assembly which will help embed the map easily
- We declare a variable, dataURL that contains the name of the Data Provider Page (WorldPopulationData.aspx)
- We pass dataURL to renderMap() function provided by the FusionMaps class of InfosoftGlobal assembly
- The XML is relayed and finally map is rendered in literal - WorldPopulationMap.
|
|
Now, let us see the code in Data Provider Page WorldPopulationData.aspx |
|
<%@ Page Language="VB" %>
<script runat="server">
Private Sub Page_Load(ByVal sender As System.Object, ByVal e As System.EventArgs)
Dim dataArray(7, 2) As String
dataArray(0, 0) = "01"
dataArray(0, 1) = "3779000000"
dataArray(1, 0) = "02"
dataArray(1, 1) = "727000000"
dataArray(2, 0) = "03"
dataArray(2, 1) = "877500000"
dataArray(3, 0) = "04"
dataArray(3, 1) = "421500000"
dataArray(4, 0) = "05"
dataArray(4, 1) = "379500000"
dataArray(5, 0) = "06"
dataArray(5, 1) = "80200000"
dataArray(6, 0) = "07"
dataArray(6, 1) = "32000000"
dataArray(7, 0) = "08"
dataArray(7, 1) = "179000000"
Dim strXML As New StringBuilder
strXML.Append("<map showLabels='1' includeNameInLabels='1' borderColor='FFFFFF' fillAlpha='80' showBevel='0' legendPosition='Bottom' >")
strXML.Append("<colorRange>")
strXML.Append("<color minValue='0' maxValue='100000000' displayValue='Population : Below 100 M' color='CC0001' />")
strXML.Append("<color minValue='100000000' maxValue='500000000' displayValue='Population :100 - 500 M' color='DDD33A' />")
strXML.Append("<color minValue='500000000' maxValue='1000000000' displayValue='Population :500 - 1000 M' color='069F06' />")
strXML.Append("<color minValue='1000000000' maxValue='5000000000' displayValue='Population : Above 1000 M' color='ABF456' />")
strXML.Append("</colorRange>")
strXML.Append("<data>")
Dim i As Integer
For i = dataArray.GetLowerBound(0) To dataArray.GetUpperBound(0)
strXML.Append("<entity id='" & dataArray(i, 0) & "' value='" & dataArray(i, 1) & "' />")
Next
strXML.Append("</data>")
strXML.Append("</map>")
Response.ContentType = "text/xml"
Response.Write(strXML.ToString())
End Sub
</script>
|
|
(Here we have used the same code for XML creation that we used in the dataXML example.) |
- We declare a 2-dimensional array, dataArray, to store the population data and Internal IDs for 8 continents.
- We store data in the array, the first column is used to store Internal IDs and the second column stores the population value of respective continents.
- Then we declare a variable strXML to store the XML of this map.
- After declaring the variable we set 4 color ranges to the strXML.
- Then we store the data from the dataArray to strXML by iterating through dataArray.
- Finally, we send this data to output stream without any HTML tags.
|
|
Here is the snap of the map: |
|
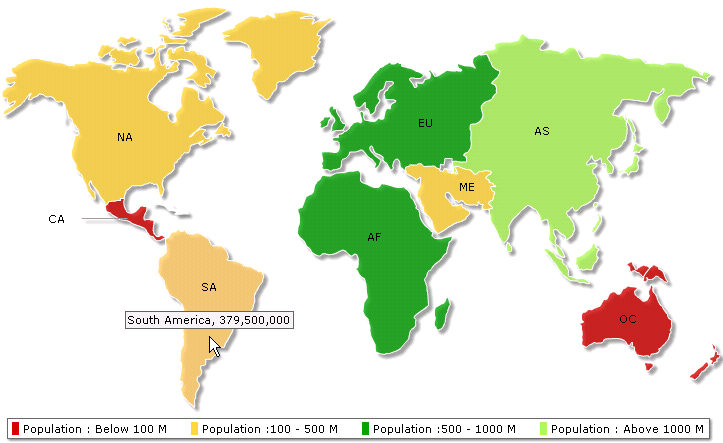 |